September 13, 2023
•4 minutes read
A Guide for Pulling Customer and Order Data from Shopify
A TypeScript Developer's Guide to Customer and Order Data
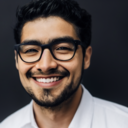
In the bustling world of e-commerce, Shopify stands out as a comprehensive platform that empowers businesses to create and manage their online stores. For developers, tapping into Shopify's vast capabilities via its API can unlock a plethora of opportunities, from customizing store functionalities to integrating with other services. TypeScript, with its strong typing and object-oriented features, is an excellent choice for building robust integrations. This guide will walk you through the process of using TypeScript to pull customer and order data from the Shopify API, ensuring type safety and efficient data handling.
Setting Up Your TypeScript Environment
Before diving into the Shopify API, ensure your development environment is
ready. You'll need Node.js and npm (or yarn) installed. Initialize a new
TypeScript project by running npm init
followed by tsc --init
to generate a
tsconfig.json
file, which you can customize for your project needs. Install
the Shopify API client library for Node.js using npm to facilitate API
interactions.
Authenticating with Shopify
Shopify's API uses OAuth for public apps and API keys for private apps. For this guide, we'll focus on a private app scenario, which is simpler and suitable for internal tools or custom integrations.
- Create a Private App in Shopify: In your Shopify admin panel, go to "Apps" and then "Manage private apps". Create a new private app to obtain your API key and password.
- Store Your Credentials Securely: Use environment variables to store your API
key and password. Create a
.env
file in your project root and add your Shopify credentials there. Remember to add.env
to your .gitignore file to keep your credentials secure.
Initializing the Shopify API Client
With your credentials stored securely, it's time to initialize the Shopify API
client. Create a new file, shopifyClient.ts
, and set up your client as
follows:
import Shopify from 'shopify-api-node';
import dotenv from 'dotenv';
dotenv.config();
const shopify = new Shopify({
shopName: process.env.SHOP_NAME,
apiKey: process.env.API_KEY,
password: process.env.PASSWORD
});
export default shopify;
This client will be used to make API calls to Shopify.
Fetching Customer Data
To fetch customer data, you'll create a function that uses the Shopify client. Define a TypeScript interface to ensure the data structure is consistent:
interface Customer {
id: number;
email: string;
first_name: string;
last_name: string;
// Add other fields as needed
}
async function fetchCustomers(): Promise<Customer[]> {
const customers = await shopify.customer.list();
return customers;
}
This function returns a promise that resolves to an array of customers, adhering
to the Customer
interface.
Fetching Order Data
Fetching order data is similar to fetching customer data. Define an interface for orders and create a function to retrieve them:
interface Order {
id: number;
total_price: string;
created_at: string;
line_items: Array<{
title: string;
quantity: number;
}>;
// Add other fields as needed
}
async function fetchOrders(): Promise<Order[]> {
const orders = await shopify.order.list({ limit: 250 });
return orders;
}
This function leverages the Shopify client to fetch orders, returning a promise
that resolves to an array of orders matching the Order
interface.
Handling Pagination
Shopify's API paginates large datasets. When fetching customers or orders, you may need to handle pagination to retrieve all records. Shopify's Node.js library simplifies pagination, but you should be aware of it when designing your data retrieval logic.
Conclusion
Integrating Shopify with TypeScript offers a powerful way to interact with your Shopify store programmatically, enabling custom solutions that can drive business growth. By following the steps outlined in this guide, you can start pulling customer and order data from Shopify, leveraging TypeScript's strong typing system to ensure reliability and efficiency in your integration.
Remember, this guide focuses on private apps for simplicity. If you're building a public app, you'll need to implement OAuth authentication, which introduces additional complexity but also opens up your app to a wider audience.
As you become more familiar with Shopify's API and TypeScript, you'll discover even more ways to enhance your e-commerce solutions, from automating workflows to integrating with other platforms and services. Happy coding!